When you are testing In-App purchasing in your Windows 8 app, you need to use “Windows.ApplicationModel.Store.CurrentAppSimulator” static class instead of “Windows.ApplicationModel.Store.CurrentApp”. This means you’ll end up writing a lot of code like this:
var licenseInformation =
#if DEBUG
Windows.ApplicationModel.Store.CurrentAppSimulator.LicenseInformation;
#else
Windows.ApplicationModel.Store.CurrentApp.LicenseInformation;
#endif
//...
string receipt = await
#if DEBUG
Windows.ApplicationModel.Store.CurrentAppSimulator.
#else
Windows.ApplicationModel.Store.CurrentApp.
#endif
RequestProductPurchaseAsync(featureName, true)
Yes this gets ugly really quick. Here’s a neat little trick to get around that. Right below your using statements at the top of your file, simply add this alias mapping:
#if DEBUG
using Store = Windows.ApplicationModel.Store.CurrentAppSimulator;
#else
using Store = Windows.ApplicationModel.Store.CurrentApp;
#endif
And in your code you can now simply write:
var licenseInformation = Store.LicenseInformation;
string receipt = await Store.RequestProductPurchaseAsync(featureName, true);
With the new Windows Phone 8.0 OS a lot of things has changed with the new kernel and CLR, and in addition there are quite a few breaking changes to the SDK. However WP8 has a “quirks mode” that it uses to detect if an app was built for 7.1 (Mango), it will execute it as if it was running on a WP7.1 device. That means that if your app is using some of the features that has changed in WP8, it should continue to run with no problems (I have identified several compatibility issues though). This is great because the over 120,000 apps in the store today should (for the most part) work on your new WP8 device.
However if you upgrade your app and compile it for WP8, you will exit this quirks mode, and your app could potentially break if you are subject to any of the breaking changes. You can find a good list of the breaking changes here: http://msdn.microsoft.com/en-US/library/windowsphone/develop/jj206947(v=vs.105).aspx
Upgrading poses a big problem though: Most of you are probably relying on 3rd party libraries that haven’t been upgraded to or certified for WP8. The Quirks Mode is enabled for your entire app, and cannot run parts of your app in quirks mode, and other parts outside quirks mode. This means if your 3rd party library hits any of these breaking changes and you use it in a WP8 app, your app WILL break.
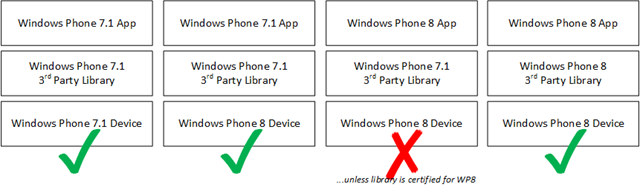
If you are a 3rd party library developer, you should test your library for compatibility. If you find any issues, you should probably release two versions, one for WP7 and one for WP8.
Of course if you don’t really need any of the new WP8 features, my recommendation is to stick to WP7.1; - at least until there’s a big enough user-base for WP8 and a small WP7.1 user-base.
Note: All this also applies to good old browser Silverlight which have had quirks mode for a long time, so this isn’t a new concept. This is actually the reason that the product I work on releases both Silverlight 4 and Silverlight 5 versions, because the Silverlight 4 assemblies causes problems when used in a Silverlight 5 app. We had a lot of customers still stuck on SL4 and others who wanted to use new SL5 features, so we chose to support both for some time. The same will be the case for WP7 which will be around for a while, and you might have to support both.